Piece Movements in Chesslike - Chess vs Chesslike
When I first had the idea of putting chess pieces in levels that weren't chess boards, I thought it would be an easy way for me to learn some programming given the plethora of chess tutorials and articles online. I also wanted to do something to study and practice level design and puzzles in levels. So the level editor was created first, because it was easier for my junior brain to handle as I developed my thoughts on chess movements and chess AI.
Normal chess movement calculations and ai are based on an 8x8 grid, and that never ever changes. It's a convenient number, 64, and with the limited number of types of pieces, folks actually save the board as a 64bit bitboard. Each bit represents what type of piece is in that spot on that board. Each of those types have a score associated with them as well.
Add your piece scores, and subtract the enemy pieces scores, and you get a board score. Then on the enemy's turn, they do the same but adding their scores and removing yours. This is the basis on the naming for a common chess AI, Minimax. Here's a nice little overview from geeksforgeeks.org:
In Minimax the two players are called maximizer and minimizer. The maximizer tries to get the highest score possible while the minimizer tries to do the opposite and get the lowest score possible.
Every board state has a value associated with it. In a given state if the maximizer has upper hand then, the score of the board will tend to be some positive value. If the minimizer has the upper hand in that board state then it will tend to be some negative value. The values of the board are calculated by some heuristics which are unique for every type of game.
In chess that heuristic is the board score, made up of piece scores. Piece scores are somewhat debated, but a good starting point could be:
- Pawn: 1
- Knight: 3
- Bishop: 3
- Rook: 5
- Queen: 9
- King: 12-15, infinity?
The pawns are the weakest, the knights and bishops score about the same apparently, the rook slightly higher, the queen a lot higher, and the king is basically anywhere from more than the queen, to infinity.But if the king is captured the game is won—that should outweigh literally anything else. It's here that Chesslike begins to break away from traditional chess.
Our Differences
In Chesslike, and soon-to-be-released Super Chesslike, we have a few differences from the original game of chess, all of which impact how we calculate legal moves, which in turn affects the AI, and many other systems within the game.
In other words, this is where the fun begins.
The very first thing is the board. I basically want the board to be anything but 8x8. That's the original hook of the game. Chess pieces, not on chess boards. You can probably already imagine some boards in your head that might be fun. Once you start test these out, you run into a few issues with using the traditional chess ruleset. Let's go over some of those issues, and discuss how Chesslike has chosen to deal with them.
Pawns
Pawns only move in one direction in chess. Any level that's not unidirectional quickly faces this issue with pawns. When we create puzzles on levels, it may became unclear which way a pawn should move if it were to only move in 1 direction. We could give boards a direction, but that seemed pretty restraining, and so we thought it might be more fun to allow pawns to move in 4 directions all the time.
We maintain the attack pattern by allowing the pawns to attack diagonally only. So they can move in four directions, and attack in four other directions. Pawns already were the only pieces that could move to some spaces and only attack different spaces. All other pieces can move and attack the same spaces. Pawns are very unique in that way.
We opted not to allow for En Passant as it is sort of complicated and breaks a more elegant simpler set of move rules for pawns which we felt lends better toward a more general audience, rather than chess enthusiasts. We also took away the pawns ability to move 2 squares in its first move, but we may bring that ability back still. It was present in the first game. All of these changes should give the pawn's score a little boost. So we say it's a 2 now. It has more opportunity, but it's still pretty limited.
Knights & Valid Paths
Next up is the knight. A knight's movements are basically the same in Chesslike as they are in regular old chess. There is one slight difference. When a chessboard is setup, the knights are on the back row and what makes them special is that in their movement, they can sort of "jump" over other pieces to get to their destination. This means you can move them on their first turn from behind the initial starting wall of pawns. It's definitely a cool mechanic that we wanted to maintain in Chesslike.
But we also have things like holes in the ground, or walls that should be impassable. You might think the knight should be able to "jump" over those holes, or perhaps over the walls as well. However, we found that when designing our levels, our canonical assumption of the pieces movements were that it could not traverse those tiles in the board.
So we need to validate the path from the knight's starting position to its destination, to guarantee that it is not jumping a hole, or wall, or passing through a locked door. That classic "L" shaped movement path actually means there are two different potential paths to a single spot. We need to have at least one of them be valid in order for the knight to have a legal move there. Knights can still jump over other pieces and items though.
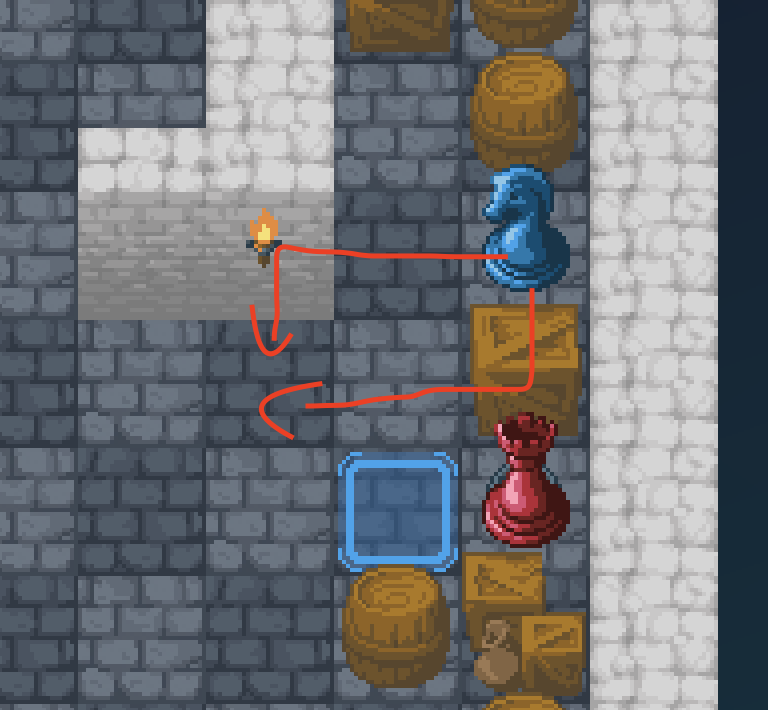
So to determine if a knight has a valid path, we store the two paths along with each destination. The destination above would be [-2, 1], that is the relative distance from it's start to it's destination. left 2 tiles, down 1 tile, or down 1 tile, and left 2 tiles. In code that looks like this:
{ dest: [-2, 1], alpha: [ [-1, 0], [-2, 0] ], beta: [ [0, 1], [-1, 1] ] }
When checking each of the knight's move possibilities, we pass the relative tiles from each path to the isValidPath function below to make sure the knight can make it to that spot via at least one of the two. This will be run for both alpha and beta paths above to reach the destination. It checks for unwalkable tiles (walls, level obstructions, holes), or locked doors at the moment:
export function isValidPath(relativeTiles, origin, map, mapWidth) { let valid = true; relativeTiles.forEach(tile => { const { logicTile } = getTileLayers(tile, origin, map, mapWidth); if (logicTile) { // prevent passing through unwalkable tiles if (logicTile.index === UNWALKABLE) { valid = false; } // prevent passing through locked doors if (logicTile.index === LOCKED_DOOR) { valid = false; } } }); return valid; }
Items/Interactables
Chesslike adds a few extra things to the boards to allow for more puzzle and strategy opportunities which have affects on how pieces move. In the first Chesslike game we added swords and shields as ways to upgrade pieces dynamically. We also added warps to magically move pieces from one tile to another across the board. There are keys, and locked doors. We've kept all of these things in the new game, with some slight modifications. Weapon upgrades will be handled differently, but in terms of how pieces move around them, it is the same. The enemy pieces cannot move onto tiles with items, and treats them as unwalkable tiles when determining moves via AI. We're also planning to add a few more new items, or environmental entities. Stay tuned...
Kings Are Useless, We Don't Need Them
One of the big things about Chesslike is that we don't really adhere to the goal of capturing the king. The idea in our original Chesslike game used to be that you could upgrade all the way up to a king if you used enough items on a single piece. And so the battle wasn't over if your king fell, since another piece could rise to power as a new king. And so capturing the king was no longer the goal.
It touches on something that always bothered me playing chess as a child, is that the goal is to check or checkmate the king, but then you never actually capture it to win. You just get it to the point where you will win if you keep playing, but then you don't. It sort of robs the winner of the satisfaction of knocking the king over! ...or taking it off the board gently. Furthermore, what about after that move? Some games may have ended in a way that there are lots of pieces left, and one player may still be able to win without a king. Chess just isn't set up for that sort of play though. For example, it's prone to stalemates when there are fewer pieces. We'll talk about that some more in later articles.
So the point of the game isn't to capture the king, and the game isn't won or lost by capturing, or losing a king. Actually few levels will contain a king for those reasons as well. The king's score shouldn't be the ultimate decider of winning and losing, so we need to sort of re-evaluate the king's score. As a rough estimate, we've gone with a 5, which is equal to our Bishops. It's more powerful than a pawn surely, as it can move and attack in all directions. But not as powerful as pieces that can move infinitely in a direction. So it sits higher than a pawn or knight, equal to a bishop, but less than a rook, and certainly much less than a queen.
The piece scoring has an actual algorithm in Super Chesslike, but the scoring we used for the basis, and in the original Chesslike was:
- Pawn: 2
- Knight: 4
- Bishop: 5
- Rook: 7
- Queen: 10
- King: 5
Chesslike is not chess, it's more fun than that
With Chesslike's adjusted ruleset there are countless new options and opportunities for more fun using the classic pieces we've grown to love for centuries. You can see some of what's described above in the original Chesslike still online today at chesslike.net. For the new game we're planning to dive deeper into some of these possibilities and create a longer-lasting play experience to be shared with others.
Super Chesslike Adventure
Open-world followup to Chesslike: Adventures in Chess. Join Fayette on their journey for justice across the realm.
Status | In development |
Authors | Adam Moore, Loxmyth |
Genre | Strategy |
Tags | 2D, Chess, chesslike, Open World, Pixel Art, Tactical RPG, Turn-based Strategy |
Languages | English |
More posts
- New Item System now online!Jun 29, 2021
- Super Chesslike Adventure is now on Steam!Jun 27, 2021
- Official Chesslike Discord Launched!Nov 22, 2020
- Public Alpha - Play Now!Sep 13, 2020
- Piece Movements in Chesslike - Part 2: Calculating Movements and the 5x5 GridJun 19, 2020
- Here We Go Again, or A Fresh StartMay 29, 2020
Leave a comment
Log in with itch.io to leave a comment.